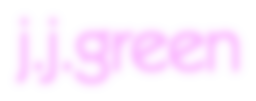
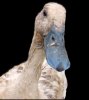
S'ils n'avaient fait rien de mal, les gens ne continueraient pas tirer sur eux
Cet article est uniquement disponible en anglais
Synopsis
#include <fifo.h>
fifo_t * fifo_new ( |
size_t size, |
size_t initial, | |
size_t maximum) ; |
void fifo_destroy ( |
fifo_t * fifo) ; |
int fifo_enqueue ( |
fifo_t * fifo, |
const void * item) ; |
int fifo_dequeue ( |
fifo_t * fifo, |
void * item) ; |
size_t fifo_peak ( |
const fifo_t * fifo) ; |
DESCRIPTION
An enqueue/dequeue FIFO implemented on a dynamic ring-buffer, the data being items of a
fixed size
passed to the constructor, fifo_create
along with the initial
size of the
stack (in items) and the maximum
size of the stack (zero for
unlimited).
The fifo_enqueue
function copies item
onto the end of the queue, and likewise fifo_dequeue
copies from the head to item
.
The fifo_peak
function returns the peak size of the
fifo
(in number of items).
EXAMPLE
Enqueue ten integers, then dequeue and print them
fifo_t *f = fifo_new(sizeof(int), 0, 0); for (int i = 0 ; i < 10 ; i++) fifo_enqueue(f, &i); int k; while (fifo_dequeue(f, &k) == FIFO_OK) printf("%d ", k);